Learn to Build Hangman in Python

The following is an excerpt from The Self-Taught Programmer: The Definitive Guide to Programming Professionally that teaches you how to build Hangman in Python.
In this chapter, you are going to combine the concepts you’ve learned so far and build a text-based game, the classic Hangman. If you’ve never played Hangman, here’s how it works:
1. Player One picks a secret word and draws a line for each letter in it (you will use an underscore to represent each line).
2. Player Two tries to guess the word one letter at a time.
3. If Player Two guesses a letter correctly, Player One replaces the corresponding underscore with the correct letter. In this version of the game, if a letter appears twice in a word, you have to guess it twice.
OR
If Player Two guesses incorrectly, Player One draws a body part of a hanged stick figure (starting with the head).
4. If Player Two completes the word before the drawing of the hangman is complete, they win. If not, they lose.
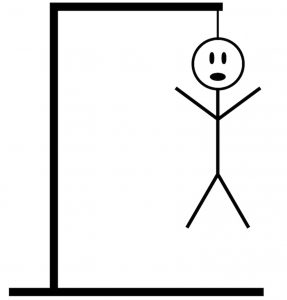
In your program, the computer will be Player One, and the person guessing will be Player Two. Are you ready to build Hangman?
Here is the beginning of your Hangman code:
# https://tinyurl.com/jhrvs94 def hangman(word): wrong = 0 stages = ["", "________ ", "| ", "| | ", "| 0 ", "| /|\ ", "| / \ ", "| " ] rletters = list(word) board = ["__"] * len(word) win = False print("Welcome to Hangman")
First, you create a function called hangman to store the game. The function accepts a variable called word as a parameter; this is the word Player Two has to guess. You use another variable, wrong, to keep track of how many incorrect letters Player Two has guessed.
The variable stages is a list filled with strings you will use to draw your hangman. When Python prints each string in the stages list on a new line, a picture of a hangman forms. The variable rletters is a list containing each character in the variable word that keeps track of which letters are left to guess.
The variable board is a list of strings used to keep track of the hints you display to Player Two, e.g., c__t if the word is cat (and Player Two has already correctly guessed c and t). You use [“__”] * len(word) to populate the board list, with an underscore for every character in the variable word. For example, if the word is cat, board starts as [“__”, “__”, “__”].
You also have a win variable that starts as False, to keep track of whether Player Two has won the game yet. Next, you print Welcome to Hangman.
The next part of your code is a loop that keeps the game going:
while wrong < len(stages) - 1: print("\n") msg = "Guess a letter" char = input(msg) if char in rletters: cind = rletters \ .index(char) board[cind] = char rletters[cind] = ' Once you are inside your loop, print a blank space to make the game look nice when it prints in the shell. Then, collect Player Two's guess with the built-in input function and store the value in the variable guess.
if not win: print("\n" .join(stages[0: \ wrong])) print("You lose! It was {}." .format(word))
Here is your complete code:
# https://tinyurl.com/h9q2cpc def hangman(word): wrong = 0 stages = ["", "________ ", "| ", "| | ", "| 0 ", "| /|\ ", "| / \ ", "| " ] rletters = list(word) board = ["__"] * len(word) win = False print("Welcome to Hangman") while wrong < len(stages) - 1: print("\n") msg = "Guess a letter" char = input(msg) if char in rletters: cind = rletters \ .index(char) board[cind] = char rletters[cind] = '
If you want to learn how to build more Python programs like this, you can buy my book and course. You can also check out One Month Python, another great resource for learning Python.
Best of luck learning Python!