How to Write Clean Code
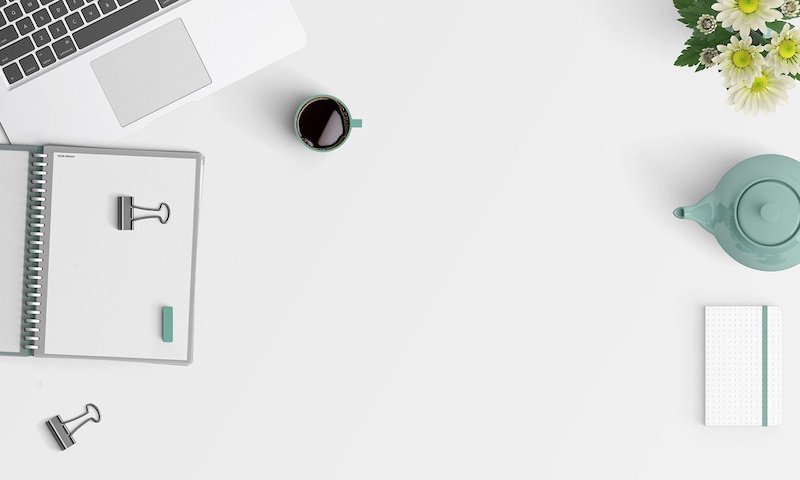
All new programmers start by writing bad code. One of the problems with teaching yourself how to code outside of school is you may not be getting the feedback you need to improve and write clean code.
Don’t worry, though; I’m here to help. Today, I’m going to give you six tips to help you write better code so that you can improve as a programmer.
Why Write Clean Code?
You might be wondering why you should bother learning to write clean code. You may be thinking, “My code works, why does it matter how it looks?”
There are a few reasons why it is essential. First of all, when you write clean code, you are less likely to introduce bugs into your program. Also, poorly written code takes longer to debug, which wastes the time of whoever has to fix it.
Finally, employers know all of this, which is why they want developers that write excellent programs. So if you can’t, it will affect your job prospects.
With that said, here are my top tips for writing clean code.
Tip 1. Follow conventions.
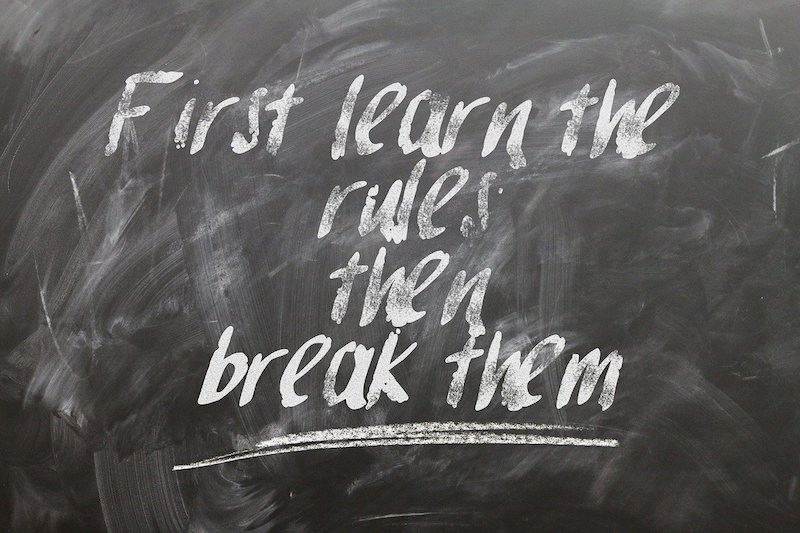
Programming languages usually have a set of conventions. Conventions are a set of guidelines that help you write better code. For example, Python has PEP-8, a set of guidelines to help you write Pythonic programs.
One of the guidelines is you should always name classes using capital letters LikeThis. Your program will still work if you name a class like_this, but your inexperience will be obvious to another Python programmer.
Python isn’t the only programming language with a written document outlining its conventions.
Google has a Java and a JavaScript style guide.
And here is one for Ruby and Swift.
Tip 2. A function or method should only do one thing.
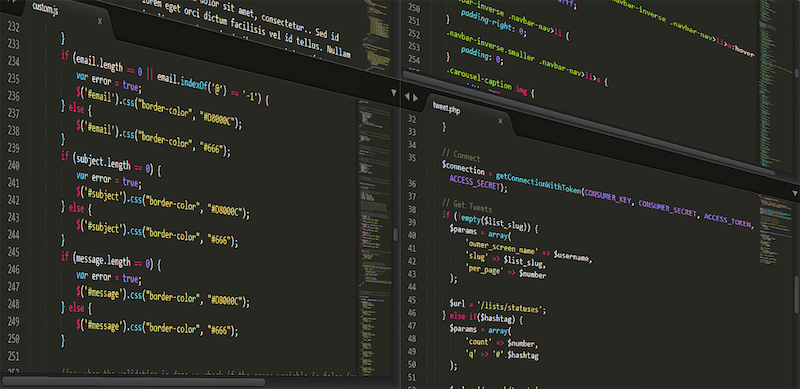
Your functions and methods should only do one thing.
Limiting your functions to one task makes your code easier to read because the person reading it can quickly figure out what your function does by reading the function name without having to read through your code and figure out the five other things it does.
When your functions only do one thing, they are short. If you are reading through your code, and see a function or method longer than 35 lines, that is a sign it is probably doing more than one thing, and you should break it up into multiple functions.
Tip 3. Edit code like an essay.
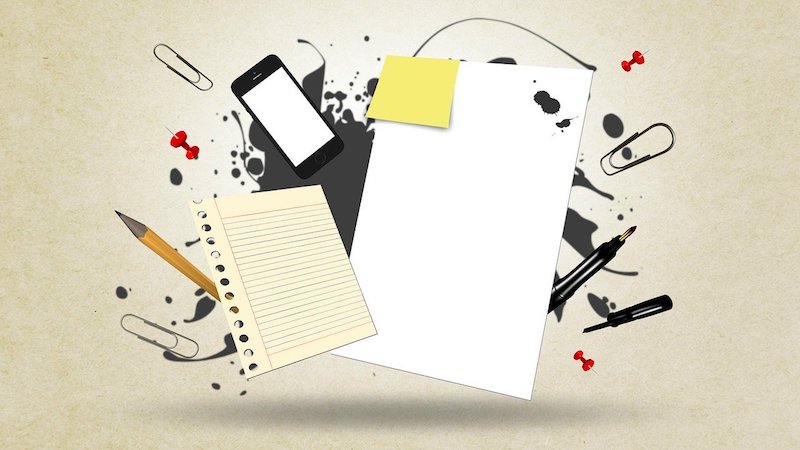
Good code is like good writing.
The key to creating a great piece of writing is your editing process. When you edit your writing, you should scrutinize every word to see if it is necessary or not. For example, I could have cut “or not” from my previous sentence and still expressed myself just as well.
You should go through the same editing process when you write code. Scrutinize each line of code. Can you express the same logic more concisely? Is there anything you can delete?
Programmers call this process refactoring, and it is what turns average code into great code.
Tip 4. Think about data structures
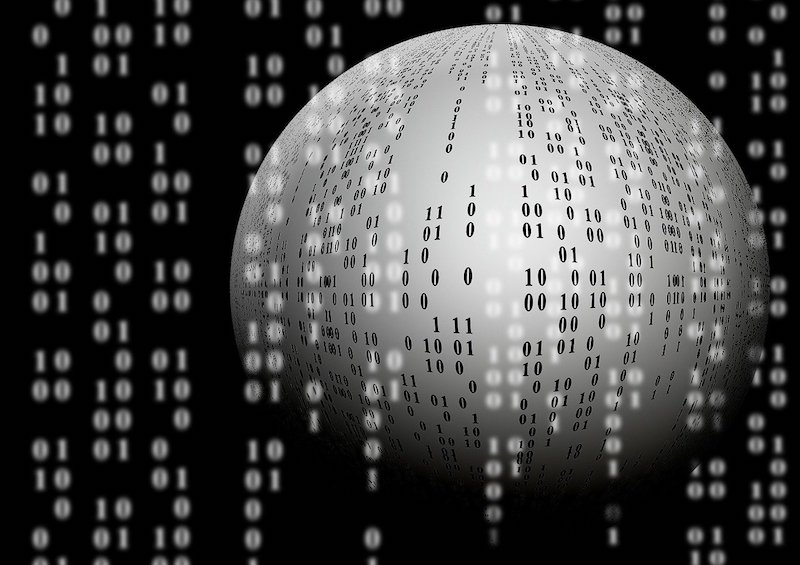
One of the assignments I give in The Self-Taught Programmer course is to build Rock, Paper, Scissors.
Most of the time, students submit Rock, Paper, Scissors code with twenty or more if/else statements. When I give feedback on this assignment, I ask if there is a data structure they could use to represent the relationships between rock, paper, and scissors?
With help, students usually realize they can map the relationship using a dictionary (in Python), and they return with a program about a third of the size.
Bad programmers dive right into writing code. Great programmers spend time planning and thinking about the data structures they will use.
Linus Torvald said it best,
I will, in fact, claim that the difference between a bad programmer and a good one is whether he considers his code or his data structures more important. Bad programmers worry about the code. Good programmers worry about data structures and their relationships.
Tip 5. Use comments but only to explain something not obvious
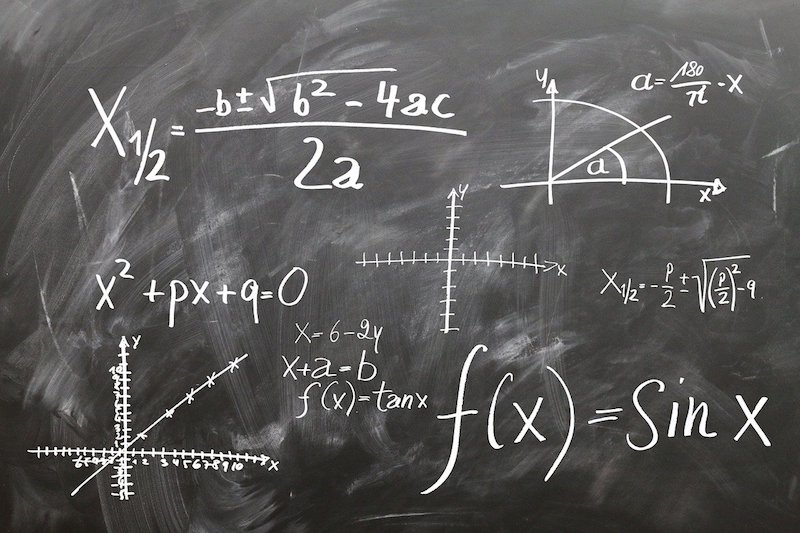
Comments can be the finishing touch on excellent code, or they can make it worse.
If you aren’t familiar with comments, they are notes your computer ignores when it runs your program that helps future programmers read and understand your code.
New programmers tend to leave too many comments. You should not put a comment in your code to explain something obvious, like that you are using a loop.
Instead, comments should explain anything that wouldn’t be immediately clear to another developer reading your code. For example, if you are using a complicated math formula, you may want to explain how it works.
Tip 6. Choose Names Carefully
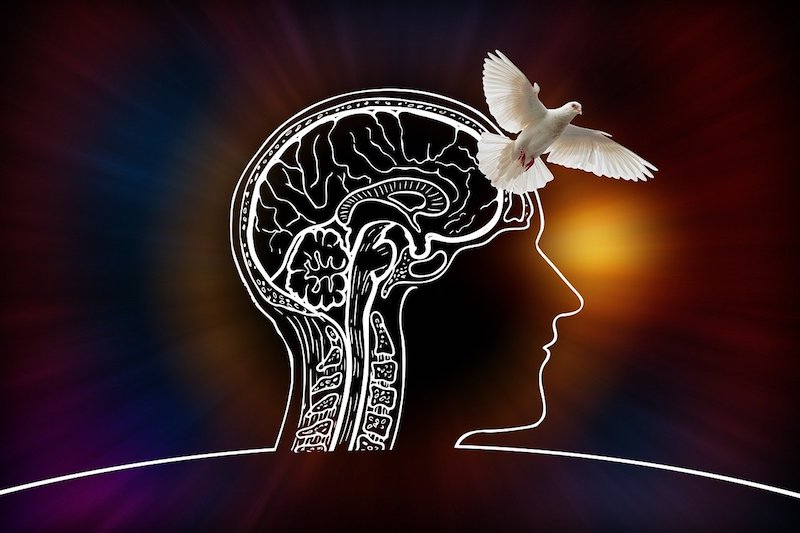
When you are programming, you are continually coming up with names. You have to name functions, classes, variables, and more.
An easy way to improve the quality of your code is to spend extra time thinking about how you name things. When you name things with purpose, your code is easy to read, whereas when you randomly name things, you make it harder to read.
For example, which of these is easier to understand?
x = 100 sixhundred = 100
Of course, naming a variable that holds the number 100 x is much clearer than naming it sixhundred.
If you make a habit of using clear, descriptive names for the things you name, you will be surprised how much easier your code is to read.
Final Thoughts
I hope this article gave you some useful tips for writing clean code.
I’m always looking for new tips on becoming a better programmer, so let me know your best practices in the comments below.
If you are looking for more resources on improving your code, here are a few suggestions.
- Clean Code.
- Kotlin for Android & Java Developers: Clean Code on Android.
- Introduction to Java Programming: Writing Good Code.
Thanks for reading!
Cory,
I am new to coding and I am using your book, when I type in the code for printing Hello
World 100 times I get incorrect syntex I can’t figure out what I am doing wrong.
Can you give me an idea of what I am possibly doing wrong.
Thank you.
Hi David. Post your question in the Self-Taught Programmers group and make sure to use a Git Gist when you send you code. https://www.facebook.com/groups/selftaughtprogrammers/
Hello, I’ve been committing two mistakes, I usually comment everything on my code and I don’t think about which days structure would be better to perform a task, thanks to this post I’ll improve it.
Awesome, glad I could help!
What heuristics do you use for the length of a function/method body? e.g. I have heard in JavaScript that it should not be longer than 10 – 12 lines.
It is whatever feels right. There is no universal rule or anything.